Parallel C User Guide
Version 2.2.2 - July 1991
516 Pages
© 3L Ltd
Introduction
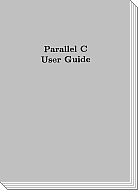
Intended Audience
This User Guide accompanies 3L's Parallel C product. It is intended for anyone who wants to use Parallel C to program a transputer system, whether writing a conventional sequential program or using the full support for concurrency which the transputer processor has to offer.
The C Language
There are two main dialects of the C language in common use: these are often referred to as "K&R C" and "ANSI C".
K&R C: This older dialect of C is defined - fairly informally - by The C Programming Language, First Edition[1], by Brian W. Kernighan and Dennis M. Ritchie, the original authors of the language.
ANSI C: This is defined, in ANS X3.159-1989[3], as the American national standard for the C language. At the time of writing, the same definition was expected to be adopted as an international standard.
The dialect of C accepted by the 3L Parallel C was originally based on K&R C. However, it has been extended by adding most of the features of ANSI C, including, for example, function prototypes and enumerated types. Details of Parallel C's ANSI extensions may be found in section 9.1.
In addition, the run-time library includes nearly all of the features of the ANSI run-time library. Traditional features have been retained as well, for compatibility with other compilers. To this have been added functions providing control of the transputer's special features, such as channel communications, concurrent execution threads, and so on.
Hardware Assumptions
Parallel C can be used with a large variety of development and target transputer systems.
The compiler itself and all the supporting utilities run on a T414 or T800 processor. This manual makes the simplifying assumption that the development environment will be an Inmos IMS B004 transputer evaluation board, or a transputer system which is largely compatible with a B004. This board is a single plug-in card for the standard IBM PC bus, with one transputer and either 1 or 2MB of RAM.
The assumption is also made here that the host computer for the B004 will be an IBM PC with a hard disk drive, or one of the many personal computers compatible with the original IBM machines.
A variety of target processors are supported by Parallel C.
- The T414 and T800 target environment is assumed to be similar to the development environment described above. Both processors are fully supported by Parallel C. However, early pre-production transputers contained faults which may cause problems with the operation of Parallel C programs. If you will be using early transputer chips, you should check appendix B for details of the problems which you may encounter, and how to get round them.
- The T425 processor can be used with Parallel C if it is treated as if it were a T414; some additional instructions are included in this processor which are not at present accepted by the in-line assembler within Parallel C. If you wish to use these instructions in assembly-language code, you must code them using the opr instruction instead.
- Parallel C can also be used to build programs for the 16-bit T212 and T222 processors. Target environments for these are discussed in chapter 8.
Document Structure
There are four main divisions within this document, as follows:
- Part I: Getting Started covers installing Parallel C on your machine and verifying that it is operating correctly.
- Part II: Tutorial introduces you to the operation of the compiler and the other tools supplied with Parallel C. In particular, there are tutorial sections explaining parallelism on the transputer and the way in which this can be accessed from Parallel C programs.
- Part III: Reference contains the detailed technical information which you will require to write sophisticated applications for the transputer using Parallel C.
- The appendices at the end of this manual contain supplementary information in a condensed form, such as tables of transputer assembly language mnemonics.
Further Reading
This User Guide does not attempt to teach the C language itself; rather, reference should be made to one of the many introductory texts available. The first - and still one of the best - books about C is the original book describing the language. This is The C Programming Language, First Edition[1], by Brian W. Kernighan and Dennis M. Ritchie.
As Parallel C includes so many ANSI features, it may be useful to consult the second edition[2] of this book, by the same authors, which describes the standard dialect. However, as certain ANSI features are not supported by Parallel C, beginners in particular may find the first edition preferable. Both editions are available in most bookshops or from the publishers.
The reader is assumed to be reasonably familiar with the operating system of the host computer being used. For personal computers made by IBM, this will usually be PC-DOS, which is supplied with a manual called Disk Operating System Reference[4]. For compatible machines made by other manufacturers, the operating system will usually be MS-DOS, described in Microsoft MS-DOS User's Reference[5]. These two operating systems are largely compatible, and their documentation is very similar. We will refer to "MSDOS" in this manual to mean the operating system used on your machine. The term DOS Reference Manual will be used to refer to the appropriate manual.
References to these and other documents mentioned in this manual are collected in a bibliography, which can be found on page 487.
Conventions
Text Conventions
Throughout this manual, text printed in this typeface represents direct verbatim communication with the computer: for example, pieces of C text, commands to MS-DOS and responses from the computer.
In examples, text printed in this typeface is not to be used verbatim: it represents a class of items, one of which should be used. For example, this is the format of one kind of compilation command:
t8c source-file
This means that the command consists of:
- The word "t8c", typed exactly like that.
- A source-file: not the text source-file, but an item of the source-file class, for example "myprog.c".
Installation Directory
As we shall see in chapter 1, it is possible to install the Parallel C compiler and its associated software in any directory. By default, however, it will be installed in directory \tc2v2, and throughout the rest of the User Guide it will be assumed that this is what has been done. Users who have chosen to install the software in another directory should replace the directory \tc2v2, wherever it is mentioned, by the name of their own installation directory.
Contents
Introduction Intended Audience The C Language Hardware Assumptions Document Structure Further Reading Conventions Text Conventions Installation Directory 1 Installing the Compiler 1.1 Installation Directory 1.2 Installing the Software 1.3 The Search Path 1.4 Environmental variable 3LCC_INC 2 Confidence Testing 3 Developing Sequential Programs 3.1 Editing 3.2 Compiling 3.3 Linking 3.3.1 Linking More than One Object File 3.3.2 Indirect Files 3.3.3 Calling the Linker Directly 3.3.4 Libraries 3.4 Running 3.4.1 Using C Programs as MS-DOS Commands 3.4.2 Command-Line Arguments 3.4.3 I/O Redirection and Piping 3.5 Memory Use 3.5.1 Default Memory Mapping 3.5.2 Alternative Memory Mapping 3.5.3 Limit on Program Memory 4 Introduction to Parallel C 4.1 Abstract Model 4.2 Hardware Realisation 4.3 Software Model 4.4 Simultaneous Input 4.5 Parallel Execution Threads 4.6 Configuring an Application 4.7 Processor Farms 5 Developing Parallel Programs 5.1 Configuring One User Task 5.1.1 Hardware Configuration 5.1.2 Software Configuration 5.1.3 Building the Application 5.2 More than One User Task 5.2.1 Inter-Task Communication Functions 5.3 Building Multi-Task Systems 5.4 Multi-Transputer Systems 5.5 Simultaneous Input 5.6 Multi-Threaded Tasks 5.6.1 Creating Threads 5.6.2 Threads versus Tasks 5.7 Debugging 5.8 Estimating Memory Requirements 6 Global Input/Output 6.1 One Transputer 6.2 More than One Transputer 6.3 More than One Multiplexer 6.4 Limits 6.5 Termination of an Application 7 Processor Farms 7.1 The Worker Task 7.2 The Master Task 7.3 The net Package 7.3.1 Functions net_send and net_receive 7.3.2 The net_broadcast function 7.4 Building the Application 7.4.1 Configuration File 7.5 Running the Example 7.6 Heterogeneous Networks 8 Developing T2 Programs 8.1 Compiling 8.2 The Compiler in T2 Mode 8.2.1 Language Restrictions 8.2.2 Pre-defined Macros 8.2.3 Data-type Representations 8.2.4 Compiler Error Messages 8.3 Linking T2 Tasks 8.4 Linker Support for the T2 8.4.1 Linker Command Switches 8.4.2 The Bootstrap 8.5 The Run-Time Library 8.5.1 Functions Defined in alt.h 8.5.2 Functions Defined in chan.h 8.5.3 Functions Defined in chanio.h 8.5.4 Functions Defined in ctype.h 8.5.5 Functions Defined in locale.h 8.5.6 Functions Defined in par.h 8.5.7 Functions Defined in sema.h 8.5.8 Functions Defined in setjmp.h 8.5.9 Functions Defined in signal.h 8.5.10 Functions Defined in stdlib.h 8.5.11 Functions Defined in string.h 8.5.12 Functions Defined in thread.h 8.5.13 Functions Defined in timer.h 8.6 Running T2 Programs 8.6.1 Using the Configurer to Boot a T2 8.6.2 Piping Code into a T2 8.7 Parameters to Main
Introduction
Overview Standard Syntactic Metalanguage 9 C Compiler Reference 9.1 The C Language 9.1.1 ANSI Features 9.1.2 Special Features 9.1.3 System-dependent Features 9.2 The C main Function 9.3 Running the Compiler 9.4 Compiler Switches 9.4.1 Default Switches 9.4.2 Controlling Output Files 9.4.3 Controlling Object Code 9.4.4 Controlling Code Patch Sizes 9.4.5 Controlling Debugging 9.4.6 Controlling #include Processing 9.4.7 Macro Definitions 9.4.8 Information from the Compiler 9.5 Predefined Macros 9.6 Handling of #include Files 9.7 Assembly Language 9.7.1 When to Use Assembly Language 9.7.2 Assembly Language Syntax 9.7.3 Literal Operands 9.7.4 Variables as Operands 9.7.5 Accessing Complex Structures 9.7.6 Labels and Jumps 9.7.7 Literal Machine Code 9.7.8 Errors 9.8 Data-type Representations 9.8.1 Integral Data Types 9.8.2 Pointer Types 9.8.3 Floating Types 9.8.4 Alignment and Complex Types 9.9 Compiler Error Messages 9.9.1 Compiler Error Message Format 9.9.2 Fixing Errors Detected by the Compiler 9.9.3 List of Error Messages 9.9.4 Errors in Assembler Language 10 The C Run-Time Library 10.1 Introduction 10.1.1 Purpose of the Run-Time Library 10.1.2 Versions of the Run-Time Library 10.1.3 Conventions 10.1.4 Header Files 10.1.5 Errors <errno.h> 10.1.6 Limits <float.h> and <limits.h> 10.1.7 Common Definitions <stddef.h> 10.2 Alt Package <alt.h> 10.3 Diagnostics <assert.h> 10.4 Neighbouring Transputers <boot.h> 10.5 Channels <chan.h> 10.6 Character Handling <ctype.h> 10.6.1 Character Testing Functions 10.6.2 Character Mapping Functions 10.7 Accessing DOS Functions <dos.h> 10.8 Localisation <locale.h> 10.9 Mathematics <math.h> 10.9.1 Treatment of Error Conditions 10.9.2 Trigonometric Functions 10.9.3 Hyperbolic Functions 10.9.4 Exponential and Logarithmic Functions 10.9.5 Power Functions 10.9.6 Nearest Integer, Absolute Value and Remainder Functions 10.10 Processor Farm Communications <net.h> 10.11 Synchronising Access to Run-Time Library <par.h> 10.12 Semaphores <sema.h> 10.13 Emulating the filter Task <serv.h> 10.14 Nonlocal Jumps <setjmp.h> 10.15 Signal Handling <signal.h> 10.16 Variable Arguments <stdarg.h> 10.17 Input/Output <stdio.h> 10.17.1 Stream I/O 10.17.2 Binary I/O 10.17.3 Text I/O 10.17.4 Operations on Files 10.17.5 File Access Functions 10.17.6 Formatted Input/Output Functions 10.17.7 Character Input/Output Functions 10.17.8 Direct Input/Output Functions 10.17.9 File Positioning Functions 10.17.10 Error Handling Functions 10.18 General Utilities <stdlib.h> 10.18.1 String Conversion Functions 10.18.2 Pseudo-Random Sequence Generation Functions 10.18.3 Memory Management Functions 10.18.4 Communication with the Environment 10.18.5 Searching and Sorting Utilities 10.18.6 Integer Arithmetic Functions 10.18.7 Multibyte Character Functions 10.18.8 Multibyte String Functions 10.19 String Handling <string.h> 10.19.1 Copying Functions 10.19.2 Concatenation Functions 10.19.3 Comparison Functions 10.19.4 Search Functions 10.19.5 Miscellaneous Functions 10.20 Threads <thread.h> 10.21 Date and Time <time.h> 10.22 Transputer Timers <timer.h> 11 Alphabetic List of Run-time Library Entries 12 The Linker 12.1 Command Line 12.2 File Name Conventions 12.3 The Output File 12.4 Indirect Files 12.5 Libraries 12.6 The Executable Image 12.7 Map Files 12.8 T2 Support 12.8.1 Switch /Msize 12.8.2 Switch /Asize 12.8.3 Switches /FC, /FA, /FS, and /FH 12.8.4 Modified /F Switches 12.8.5 Switch /Rsize 12.9 Debug Tables 12.10 Summary of Switches 12.11 Using Batch Files 12.12 Duplicate Definitions 12.13 Messages 13 The mempatch Utility 13.1 Identifying mempatch 13.2 Invoking mempatch 13.3 Re-invoking mempatch 14 The decode Utility 14.1 Usage 14.1.1 Compilation for the Decoder 14.1.2 Running the Decoder 14.2 Features of the decode Program 14.3 Other Languages 15 The Worm Utility 15.1 Notes 16 The tnm Utility 17 The tunlib Utility 18 Configuration Language Reference 18.1 Standard Syntactic Metalanguage 18.2 Configuration Language Syntax 18.2.1 Low Level Syntax 18.2.2 Numeric Constants 18.2.3 String Constants 18.2.4 Identifiers 18.2.5 Statements 18.2.6 PROCESSOR Statement 18.2.7 WIRE Statement 18.2.8 TASK Statement 18.2.9 CONNECT Statement 18.2.10 PLACE Statement 18.2.11 BIND Statement 19 Flood-Fill Configurer Reference 19.1 User Task Protocol 19.1.1 Master Task's Ports 19.1.2 Worker Task's Ports 19.2 Packet Format 20 Task Data Sheets
Appendices
A Distribution Kit A.1 Directory \tc2v2 A.2 Directory \tc2v2\examples B Compatibility with T414A and T800A B.1 Problems with T414A B.1.1 Restriction on Message Lengths B.1.2 Problems with Timers B.2 Problems with T800A B.2.1 Floating-Point Conversion Problems B.2.2 Instruction Decode Problems C Building a Network C.1 Network Principles C.2 Network Requirements C.2.1 Requirements for Links C.2.2 Requirements for System Services C.3 Connecting a Network D Summary of Option Switches D.1 Compiler Switches D.2 Linker Switches D.3 afserver Switches D.4 General Purpose Configurer Switches E Transputer Instructions E.1 Pseudo-Instructions E.2 Prefixing Instructions E.3 Direct Instructions E.4 Operations E.5 T4-only Instructions E.6 T8-only Instructions E.6.1 Floating Point Instructions E.6.2 Other T8-only Instructions F Compatibility Functions F.1 Introduction F.1.1 ASCII Control Codes <ascii.h> F.1.2 Channel Communications <chanio.h> F.1.3 Variable Arguments <varargs.h> F.2 Low-Level I/O F.3 Alphabetic List of Compatibility Functions G Mandelbrot Program Listings G.1 Mandelbrot Example Master Task G.2 Mandelbrot Example Worker Task G.3 Header File G.4 Configuration File H ASCII Code Chart Bibliography Index